ScriptRunner Blog
Parameter validation concepts in PowerShell & ScriptRunner
Table of contents
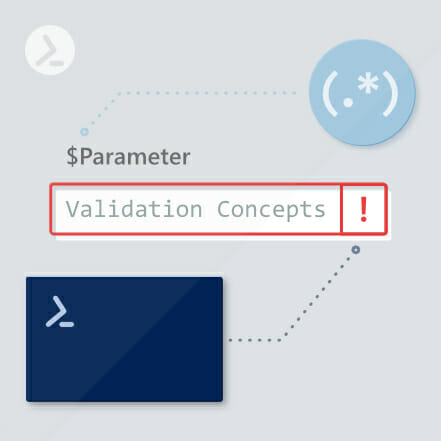
For those who often delegate (PowerShell-) tasks that require user input, there is always one factor you can’t control and that is the end-user skills: even with detailed explanation and help there can and will happen errors.
PowerShell provides a few options to validate your input and prevent input-errors. ScriptRunner processes these options and gives the end-user great visibility on the validity of the input before executing the script, next to that it will prevent the execution of the script if the criteria are not met.
In the following paragraphs, I will discuss two options to validate user input with PowerShell & ScriptRunner.
ValidateSet-attribute
The first option for validating parameters in PowerShell is the ValidateSet-attribute. It allows you to specify a set of valid values for a parameter or variable and additionally enables tab completion in the shell. This eliminates the risk of erroneous and wrong input from the en-user, since the input options are predefined.
ScriptRunner furthermore increases usability by translating this validation option to a nice drop-down menu.
Let us examine the parameter block below. We want to specify a pre-defined set of possibilities for the “memory” variable, in our example 8Gb, 16Gb or 32Gb.
Param (
[ValidateSet('8GB', '16GB', '32GB')]
[string]$Memory
)
As we can see in figure 1, ScriptRunner translates this piece of code into a drop-down list from which an end-user can comfortably select the desired value(s).

Figure 1a: ScriptRunner automatically translates the parameters which are contained in the ValidateSet-attribute into a drop-down list
If you’re interested in finding out more about how ScriptRunner turns your PowerShell scripts into a user-friendly GUIs, there’s an in-depth article on this topic on ScriptRunners’ tech blog: PowerShell parameters and their graphical representation in ScriptRunner
ValidatePattern -attribute
The ValidatePattern -attribute allows you to specify a regular expression int the parameter block of your script that is matched with the parameter or variable value your end-user enters.
PowerShell will throw an error if the content of the variable does not match the regular expression. ScriptRunner Delegate and Self-Service App will indicate the error through a red exclamation mark at the respective input element and will prevent script execution until the error is fixed.
Introduction to regular expressions (regex)
A regular expression is a sequence of characters that define a search pattern. Regex is known to be a bit tricky, so be sure to use the correct syntax. Below, you’ll find a short overview of the syntax used in our examples.
Anchors
Anchors are characters that specify the boundaries of the expression:
Placeholders
With placeholders, you describe which characters you expect your search result to match to:
Quantifiers
With quantifiers, you can specify how many times the characters defined through the placeholders are part of your pattern.
Example of a regular expression
Let’s try a simple example: we want to validate a string that only matches letters of the alphabet.
Here’s one possibility how we can achieve this through regular expressions:
^[a-z]+$
In the table below, you’ll find the expression divided into its separate parts, to make it clearer what is happening:
Regex validation in PowerShell
When the expression is shaped, we can use it to validate string variables in PowerShell.
Several PowerShell operators (e.g. -match, -split, -replace) as well as cmdlets (select-string) are supporting regex.
Keep in mind that PowerShell regex expressions are by default case-insensitive. If you want to make them case-sensitive use the ‘c’ in the operators (e.g. -cmatch, -csplit, -creplace, as seen in figure 2).
Using regex with PowerShell and ScriptRunner: first example
We’ll start with our expression from the regex-introduction. We want to validate a string that only matches the letters of the alphabet.
In our PowerShell script we define ValidatePattern as following:
Param (
[ValidatePattern(
'^[a-z]+$'
)
]
[string] $OnlyLetters
)
When running this script as an Action within ScriptRunner, the parameter $OnlyLetters can be easily validated. If the validation fails, an exclamation mark is displayed and the execute button is grayed-out. Below you can find a few validation tests (figures 3a-c):

Figure 3a: After validating the input for $OnlyLetters, ScriptRunner displays an error warning, since the input contains numbers, which are prohibited through the regular expression.

Figure 3b: After validating the input for $OnlyLetters, ScriptRunner displays an error warning, since the input contains spaces, which are prohibited through the regular expression.
Using regex with PowerShell and ScriptRunner: advanced second example
Another example from the field is validating a User login name in Active Directory.
The maximum length by design is 20 characters and a lot of characters are prohibited ( /[:;|=,+*?<>]’“.) , next to that every company is using their own naming conventions.
Here’s an example from a customer, the User login name should comply to the following rules:
- Length is 10-15 characters
- No numeric characters
- No underscores
- Only special char allowed is “-“
- Must start with u-
Now, let’s translate these requirements into a regular expression:
Param (
[Validate Pattern (
'^[u][-][a-z]{8,13}$'
)
]
[string] $UserLoginName
)
And again a bit of explanation on the syntax:
And, as you can see in figures 4 a-e, the validation works!

Figure 4a: After validating the input for $UserLoginName, ScriptRunner displays an error warning, since the input contains too few characters.

Figure 4b: After validating the input for $UserLoginName, ScriptRunner displays an error warning, since the input string doesn’t start with “u-“.

Figure 4c: After validating the input for $UserLoginName, ScriptRunner displays an error warning, since the input contains numbers.

Figure 4d: After validating the input for $UserLoginName, ScriptRunner displays an error warning, since the input contains the special character “$”.
Conclusion
As you can see, parameter validation is no rocket science at all, if you get these basics right. With just a minor time investment you will get fewer errors and save time and nerves in the long run. Of course, there are many more ways to make the use of PowerShell scripts safe and secure. You can find an overview in the free webinar Using PowerShell Scripts with more security.
Related posts
7 min read
New ScriptRunner Release Enhances Enterprise IT Automation with Better Security, Transparency and Efficiency
Feb 24, 2025 by Heiko Brenn
The latest ScriptRunner release enhances Enterprise IT automation with three powerful features: the new Approval Process
13 min read
Mastering Changelog Management with PowerShell
Jan 28, 2025 by Jeffery Hicks
Changelogs keep your software updates clear and organized. Learn the best practices for creating and managing them in...
14 min read
How to Use Winget and PowerShell for Efficient App Deployment
Dec 19, 2024 by Jeffery Hicks
Boost IT efficiency with Winget and PowerShell! Learn how to automate app installations, updates, and management...
About the author:
Bruno Buyck is the founder of Trouble Shooter a company that specializes in PowerShell automation. The past decade Bruno delivered workshops on how to manage and automate systems with PowerShell. He has hundreds of script running in production environments which results in over 34 Million script executions every year. He loves to teach and prove that PowerShell is “What The Fuck”.
Latest posts:
- New ScriptRunner Release Enhances Enterprise IT Automation with Better Security, Transparency and Efficiency
- Mastering Changelog Management with PowerShell
- How to Use Winget and PowerShell for Efficient App Deployment
- How to Leverage .NET in PowerShell for event-driven scripting
- Master PowerShell WMI: Automate system event monitoring effortlessly